Our API tips series will build on knowledge from previous posts, so be sure to check out our previous post.
A common request I’ve received from users in need of a SolidWorks macro to automate time consuming processes involves some variation of “run through all the items in my assembly and perform [some operation]”. Today, we’ll look at how to use the SolidWorks API to traverse your assembly feature tree.
To do this, we’ll use the “GetRootComponent3” method from the SolidWorks “Configuration” interface in the SolidWorks API (in this case, we’ll assume we want to traverse the “Default” configuration of an active assembly):
Dim swApp As SldWorks.SldWorks
Sub main()
Set swApp = Application.SldWorks
Dim swAssy As ModelDoc2
Set swAssy = swApp.ActiveDoc
Dim swConfig as Configuration
Set swConfig = swAssy.GetActiveConfiguration
Dim swRootComp as Component2
Set swRootComp = swConfig.GetRootComponent3(False)
TraverseAssyComponents swRootComp
End Sub
At the end of the main method above, notice that we’ve referenced a method called TraverseAssyComponents, but we haven’t defined it yet.
So let’s do that, and we’ll pass the root component as a parameter. Then, we’ll get the list of children, and assign the result to a variable of type Variant (which is a special data type in VB that can contain any kind of data except fixed-length string data), and use a ‘For’ loop to iterate through each child component:
Sub TraverseAssyComponents(swParentComp as Component2)
Dim swChildren as Variant
swChildren = swParentComp.GetChildren
Dim swChildComp as Component2
For i = 0 To UBound(swChildren)
Set swChildComp = swChildren(i)
Debug.Print swChildComp.Name
Next i
End Sub
At this point, if you like, you can copy the code from both blocks above, paste into a blank SolidWorks macro and run it on an assembly. For a visual representation of the results, the code above is simply printing out the name of each component (if you don’t see the results, you’ll need to be sure that the ‘Immediate Window’ is visible, from the ‘View’ menu).
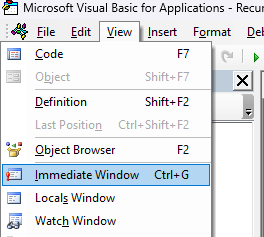
What you’ll notice is that the macro only traversed the assembly at the current level, and didn’t drill down to the child levels. To do this, we’ll use recursion. What is recursion? Well, it’s the process whereby a procedure makes a call to itself as an execution step (kind of like a snake eating it’s tail). So in our case, we’ll recursively call TraverseAssyComponents from within the For loop, passing the child component as the input parameter.
For i = 0 To UBound(swChildren)
Set swChildComp = swChildren(i)
Debug.Print swChildComp.Name
TraverseAssyComponents swChildComp
Next i
If the component has no children, the method will not enter the For loop, and will simply exit, returning to the previous recursion loop.
Here it is put all together. Give it a try for yourself!
Dim swApp As SldWorks.SldWorks
Sub main()
Set swApp = Application.SldWorks
Dim swAssy As ModelDoc2
Set swAssy = swApp.ActiveDoc
Dim swConfig As Configuration
Set swConfig = swAssy.GetActiveConfiguration
Dim swRootComp As Component2
Set swRootComp = swConfig.GetRootComponent3(False)
TraverseAssyComponents swRootComp
End Sub
Sub TraverseAssyComponents(swParentComp As Component2)
Dim swChildren As Variant
swChildren = swParentComp.GetChildren
Dim swChildComp As Component2
For i = 0 To UBound(swChildren)
Set swChildComp = swChildren(i)
Debug.Print swChildComp.Name
TraverseAssyComponents swChildComp
Next i
End Sub