We’ll start with a macro to keep things simple…
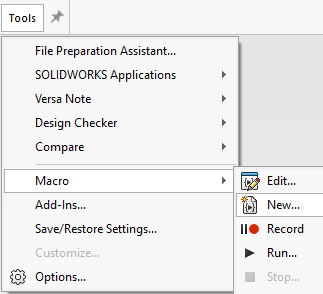
Any newly created macro will look like this:
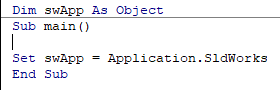
In order to take advantage of the code-completion functionality in the VBA editor, let’s swap out the generic ‘Object’ for the SolidWorks application model:
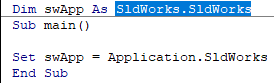
The first object we need to get is the active file, and regardless of the file type, this object will be of the ModelDoc2 type (for a really great overview, check out this post). You can see that as we type ‘swApp’ followed by a period, we can see a long list of available methods and interfaces. We want to assign the ‘ActiveDoc’ property to our new ‘ModelDoc2’ variable as follows:
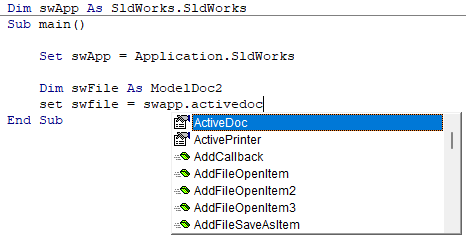
Now we can get the custom property manager, which is an interface that provides all of the read & write methods we’ll need:
Dim swApp As SldWorks.SldWorks
Sub main()
Set swApp = Application.SldWorks
Dim swFile As ModelDoc2
Set swFile = swApp.ActiveDoc
Dim swCustomPropMgr As CustomPropertyManager
Set swCustomPropMgr = swFile.Extension.CustomPropertyManager("")
End Sub
Notice that the CustomPropertyManager interface takes one input parameter a string value for the configuration name from which to read the custom property info. If we pass an empty string (i.e. double quotes), we will have access to the custom properties in the ‘Custom’ (i.e. non-configuration specific) tab.
In our case, let’s say we want to read a property called “ItemCode” in the ‘Custom’ tab of the custom property list:
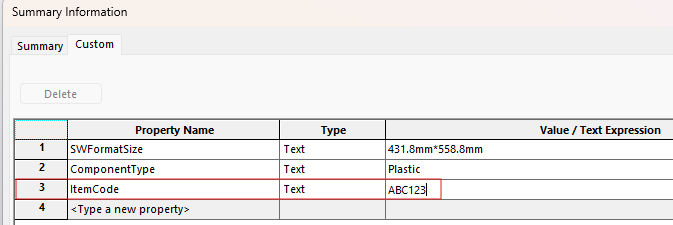
To read a specific custom property value, we’ll use the Get method. In many cases, you will notice there are multiple methods available, each with a numbered suffixes. These show the evolution of each interface throughout the SolidWorks API development history… generally speaking the highest # will give access to all of the latest & greatest SolidWorks features, but in this case I’ll use ‘Get’ for simplicity, and simply display the result in a messagebox.
Dim swApp As SldWorks.SldWorks
Sub main()
Set swApp = Application.SldWorks
Dim swFile As ModelDoc2
Set swFile = swApp.ActiveDoc
Dim swCustomPropMgr As CustomPropertyManager
Set swCustomPropMgr = swFile.Extension.CustomPropertyManager("")
MsgBox swCustomPropMgr.Get("ItemCode")
End Sub
Now to update the value, we can use the Set method, which takes 2 parameters:

Dim swApp As SldWorks.SldWorks
Sub main()
Set swApp = Application.SldWorks
Dim swFile As ModelDoc2
Set swFile = swApp.ActiveDoc
Dim swCustomPropMgr As CustomPropertyManager
Set swCustomPropMgr = swFile.Extension.CustomPropertyManager("")
MsgBox swCustomPropMgr.Get("ItemCode")
swCustomPropMgr.Set "ItemCode", "DEF456"
MsgBox swCustomPropMgr.Get("ItemCode")
End Sub
That’s it for today! Scroll down for links to follow us on the socials for more SolidWorks API tips, and for great info and a deeper dive, check out CAD Booster’s excellent summary of all things SolidWorks custom properties.